Introduction to Data Types and Variables
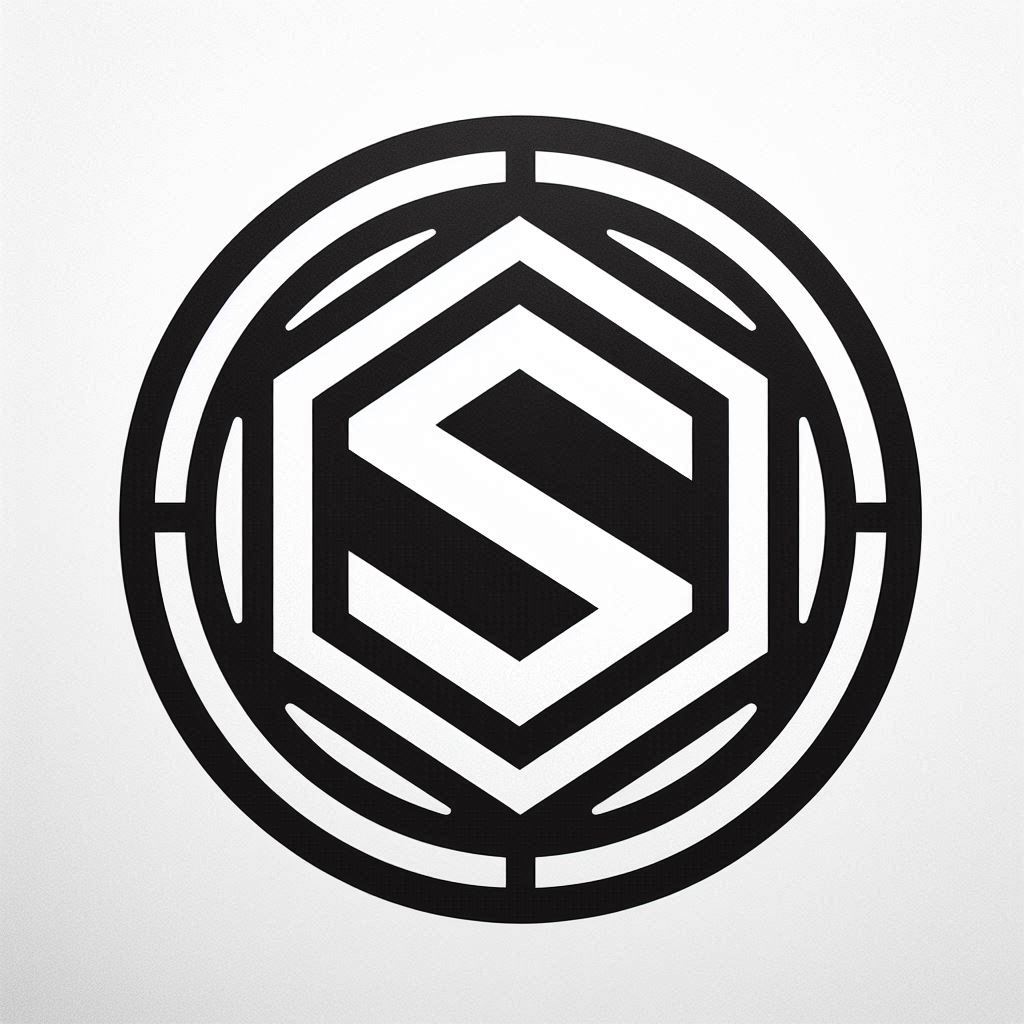
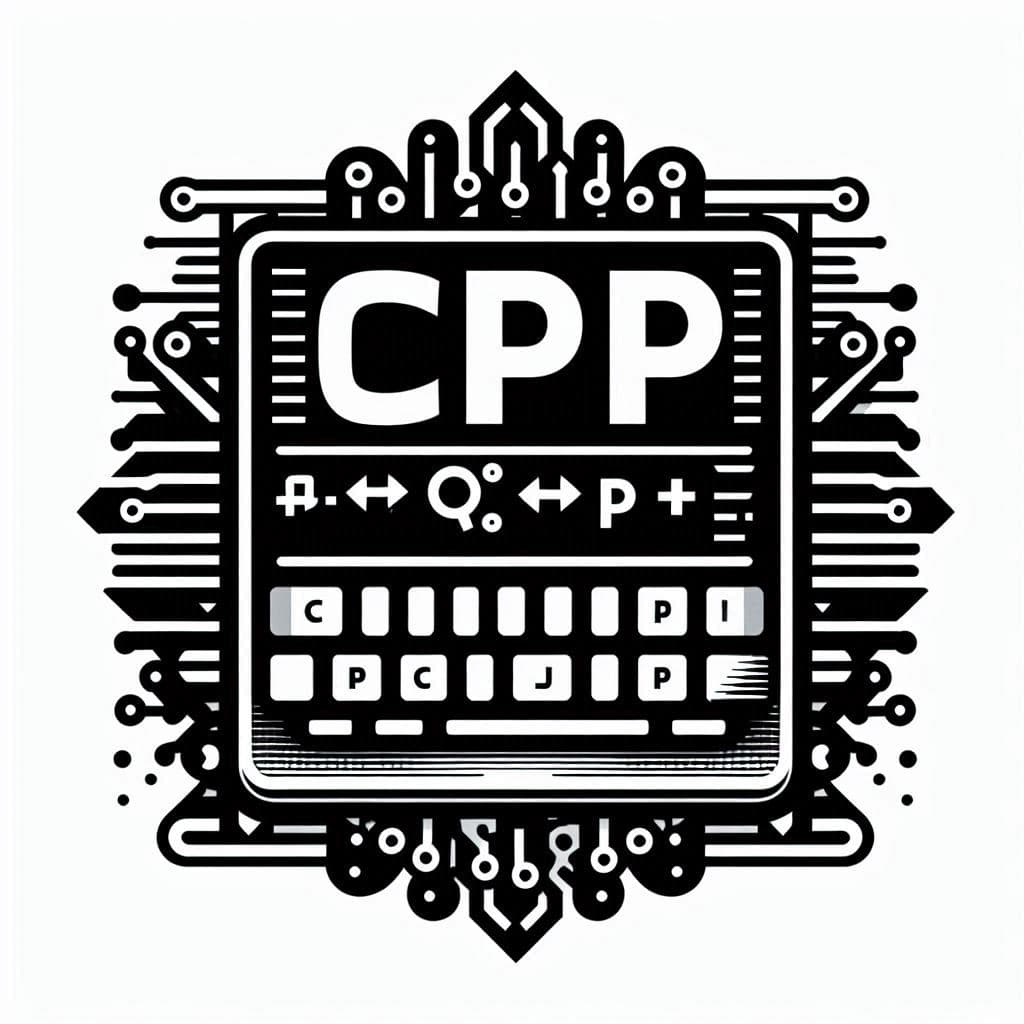
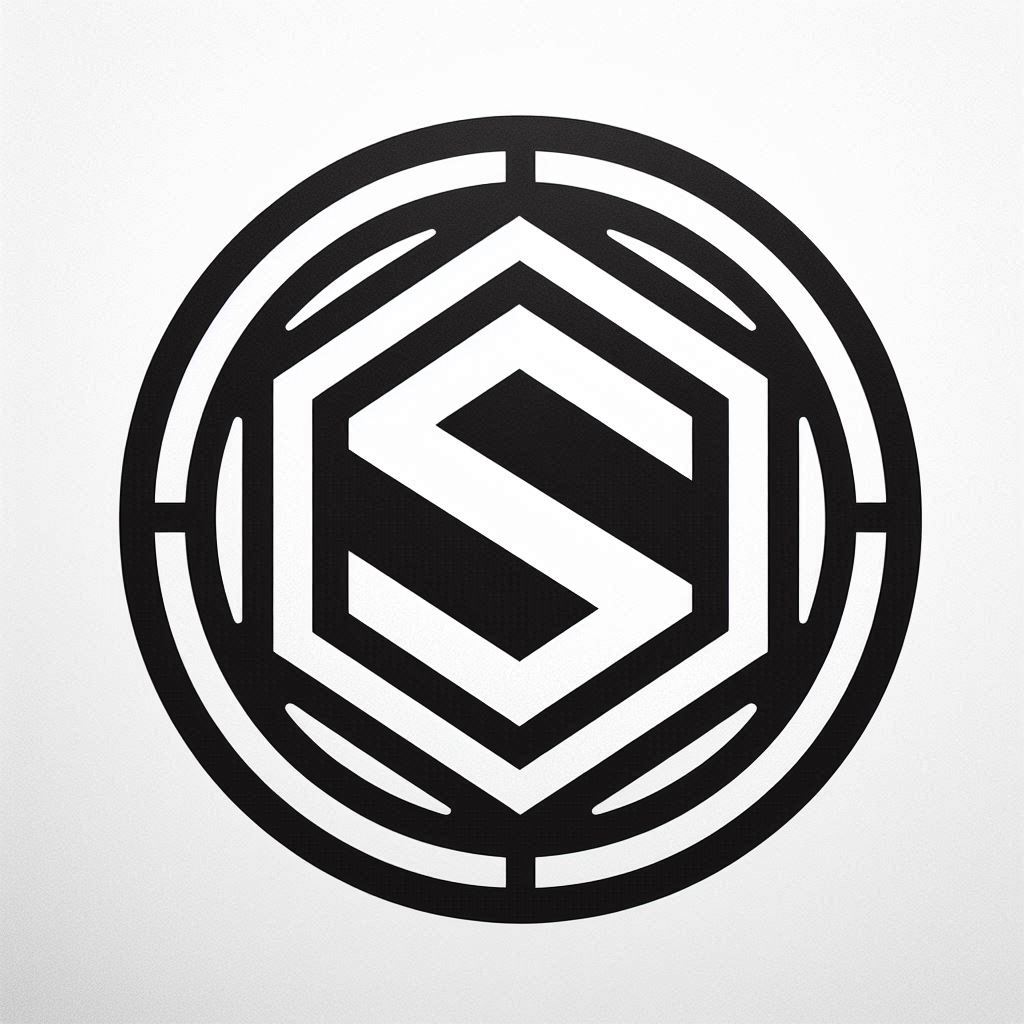
Week 2: Introduction to Data Types and Variables
"Welcome to today's lesson on Data Types and Variables in C++. In this lesson, we'll cover the basic building blocks of a C++ program—different types of data we can use, how to declare variables, and how to work with constants and literals. Understanding these concepts will be crucial as we build more complex programs in the future."
Primitive Data Types in C++
"In C++, we have several primitive data types:
- int: For whole numbers like
25
or-3
. - float: For floating-point numbers, which have decimal points like
5.99f
. - double: For double-precision floating-point numbers, offering more accuracy, like
3.14159
. - char: To store a single character, for example,
'A'
or'7'
. - bool: For boolean values, which are either
true
orfalse
.
These data types are the core building blocks for handling different types of information in a program."
Declaring and Initializing Variables
"A variable is a named storage location in memory. To declare a variable, you specify the data type followed by the variable name, like this:
int age;
float height;
char grade;
You can also initialize variables with values right away:
int age = 25;
float height = 1.75f;
char grade = 'B';
Initializing variables when you declare them helps prevent errors from uninitialized memory."
Constants and Literals
"Sometimes, we need values that won’t change during the execution of the program. These are called constants. To declare a constant, use the const
keyword:
const int MAX_SCORE = 100;
Constants like MAX_SCORE
cannot be modified later. On the other hand, literals are fixed values you use directly in your code, such as numbers (5
, 3.14
), characters ('A'
), or strings ("Hello"
)."
Code Example: Using Data Types and Variables
"Let’s see how this works in an actual C++ program. This example uses variables of different data types and outputs their values:
#include <iostream>
int main() {
int age = 30; // Integer variable
float height = 5.9f; // Floating-point variable
char initial = 'J'; // Character variable
bool isStudent = true; // Boolean variable
std::cout << "Age: " << age << std::endl;
std::cout << "Height: " << height << std::endl;
std::cout << "Initial: " << initial << std::endl;
std::cout << "Is a student: " << std::boolalpha << isStudent << std::endl;
return 0;
}
Here, we declare variables to store age, height, a person's initial, and whether they're a student, and print the values using std::cout
."
Best Practices for Variable Naming and Usage
"Here are some best practices when working with variables:
- Use meaningful names: Choose descriptive variable names like
age
,height
, andisStudent
. - Always initialize variables: Assign values when declaring variables to avoid unexpected behavior.
- Use constants for fixed values: Constants like
MAX_SCORE
make your code easier to read and maintain. - Follow naming conventions: Use lowerCamelCase for variable names (
studentAge
) and ALL_CAPS for constants (MAX_SCORE
).
Following these best practices will help you write cleaner and more reliable code."
Summary and Homework
"Today, we explored primitive data types, declaring and initializing variables, using constants, and working with literals.
For homework, try writing a program that calculates the area of a rectangle. You'll need to ask the user for the rectangle's width and height, then multiply them to get the area. Here’s a simple example to get you started:
#include <iostream>
int main() {
float width, height;
std::cout << "Enter width: ";
std::cin >> width;
std::cout << "Enter height: ";
std::cin >> height;
float area = width * height;
std::cout << "The area of the rectangle is: " << area << std::endl;
return 0;
}
Give it a try, and we’ll go over it in our next lesson."